Lesson 3.8 3.10
During this lesson sections 8 and 10 will discuss lists and iteration
- Section 8 - Iteration
- Examples
- Programming Examples
- Summary:
- HACKS Unit 3 Section 8
- Section 10 - Lists - Luna Iwazaki, Ethan Tran
- Unit 3.10.1 (Luna Iwazaki)
- Unit 3.10.2 (Ethan Tran)
- HACKS Unit 3 Section 10
Section Objectives:
- Express an algorithm that uses iteration without using a programming language
- Determine the result or side effect of iteration statements
- Write iteration statement
Section Vocabulary:
Iteration: a repeating portion of an algorithm, repeats a specified number of times or until a given condition is met
Iteration Statements: change the sequential flow of control by repeating a set of statements zero or more times, until a stopping condition is met
Repeat Until: if the condition evaluates to true initially, the loop body is not executed at all, due to the condition being checked before the loop
Unit 3 Section 8.1 - Taiyo Iwazaki
Lesson Objectives:
- Express an algorithm that uses iteration without using a programming language
- Define an iteration
- The Basics Of Iteration
- Examples
LESSON Vocab:
- Iteration: a repeating portion of an algorithm, repeats a specified number of times or until a given condition is met
Before we dive deeper, we need to understand that an iteration is a part of an algorithm. And in that iteration is a set of instructions that need to be followed until a certain desired output is met.
Examples
Here is an example using online shopping:
Lets say you are looking for a lost item inside of your house... What steps would you take?
-
Suppose there are n rooms to search... number the rooms 1-n
-
Start by searching in room number 1
-
Search each room thoroughly for you item.
-
Change the room number to the next highest number
-
Repeat steps 3 and 4 until you have found your lost item.
-
Enjoy the lost item.
WAIT! There is a flaw in the program.
This is where a stopping condition is useful for when the iteration already meets the desired requirement and gets out of the loop.
i = 0
while (i < 5):
print("Hello, World!")
i = i + 1
i = 0
while (i < 5): #Try changing the 5 and see what happens!
print("Hello, World!")
i = i + 1
if (i == 3): #Try Changing the 3 and see what happens!
break
These are just a few examples of what you can do with iteration.
Unit 3 Section 3.8.2 - Parav Salaniwal
Definition: Iteration Statement - cause statements to be executed zero or more times, subject to some loop-termination criteria
The first function we will learn is the range function, which we will use with for loop. As you may be able to guess, this will give us the sum based on the input provided. We always use a variable, such as i, to represent what the range of numbers the output will show. For example, if I wanted to list the numbers from 1-10 using the range function, it would look like this:
for i in range(10):
print(i)
Now the first thing you may notice is my input for the range was 11, and you may wonder why is it not 10? This is because when using the range function, the last number, or the ending value, is not included in the output, therefore we would have to add 1 to receive the correct output. Now if we were looking to include a starting value to have it start from 1 and end at 10, the code would look like this:
for i in range(1,11):
print(i)
When using the range with for loop, the you can list much more than just a increment of one, would anyone like to guess how we could include a change in the incremented value? Try making a change in the python code above to change the incremented value. When using the range function:
for i in range(starting value, ending value, incremented value):
print(i)
Now with while loops, we can provide a similar output with a variation in the input. Similar to for loops, it requires a variable which is the starting value.
i=1
while i<=10:
print(i)
i=i+1
Based on your knowledge from for loops, which values in the code above are the starting, incrementing, and ending values?
LESSON
Unit 3 Section 3.8.3 - Nikhil
Here is some basic code I have created, let's first look into this one: I created a list of pets, cat, dog, fish, and snake. Then I created a for loop, "for i in pets" and this looks at every element/value in pets, so "cat", "dog", "fish" and "snake". This next if statmenets checks if the element in the list is "fish" and if it is, it breaks the loop. The statement would just print, cat and dog since the loop breaks at fish.
pets = ["cat", "dog", "fish", "snake"]
for i in pets:
if i == "fish":
break
print(i)
This while loop checks if number less than 10 is even and if so, it will print out the even numbers. We set a starting value of number = 0, then while the number is less than 10, the percent sign means modulus so meaning if there is a remaninder when we dvide. So if number divided by 2 has no remainder then it will print number. Then after that it will add 1 to the number and repeat the process untill it reaches 10. So let's check for 0, while 0 is less than ten, then it moves to next statement, since 0 divded by 2 has a remainder of 0 it will print the value of 0. Now it will add 1 to zero. Then it will see that 1 is less than ten, and then check if 1 divided by 2 has a remainder of 0 which it does not, so it skips the print, and goes straight to addding one, so on so forth.
number = 0
while number < 10:
if number % 2 == 0:
print(number)
number += 1
The purpose of this code is to find the sum of all the even numbers between and any number of your choosing. The integer input asks for the minimum and maximum value. The next statement puts a starting sum value set to 0. The for i in range (minimum,maximum+1) looks at every number between those two values, in this case 1 and 100. Then the if i modulus 2 checks if any numbers that are divisible by 2 and the remainder comes to 0. If there is a number that has a remainder of 0 when divided by 2, it adds that number to the sum. Then the last print statement, prints out the final sum of all even numbers between the two numbers of your choosing.
minimum = int(input("please enter a minimum number you want your range to be"))
maximum = int(input("please enter a number that you want to be the maximum value"))
sum = 0
for i in range(minimum,maximum+1):
if i % 2 == 0:
sum += i
print("The sum of all the even numbers between", minimum, "and", maximum, ":", sum)
LESSON
- Define an Iteration Iteration is a sequence of code being repeated until a specific end result is achieved.
- Make your own example of an iteration with at least 4 steps and a stopping condition(Similar to mine that I did) When you are buying a flight ticket, you are gonna check if the price fit. If not, you will do the loop of continuous searching tickets until you find the best price. Then you are checking if the time fits, if not, search different flight until you find the best fit.
- Program a simple iteration.
bid1 = int(input("What is your initial amount"))
for i in range(0,100):
if i <= 100
- What is an iteration statement, in your own words?
- Create a descending list of numbers using for loop
- Using while loop, make a list of numbers which will form an output of 3,16,29,42,55,68,81
Section Objectives:
- For list operations, write expressions that use list indexing and list procedures
- For algorithms involving elements of a list, write iteration statements to traverse a list
- For list operations, evaluate expression that use list indexing and list procedures
- For algorithms involving elements of a list, determine the result of an algorithm that includes list traversals
Section Vocabulary:
Traversing Lists: where all elements in the list are accessed, or a partial traversal, where only a portion of elements are accessed (can be a complete traversal)
Essential Knowledge:
-
List procedures are implemented in accordance with the syntax rules of the programming language
-
Iteration Statements can be used to traverse a list
- !!! AP EXAM provides pseudocode for loops
- Knowledge of existing algorithms that use iteration can help in constructing new algorithms:
Section 10 - Lists
Since CS is taught in different languages the pseudocode represents fundamental programming concepts.
AP Exam Reference Sheet
- provides basic operations on lists
- provides pseudocode for loops
- helps you understand code used in questions
- students can use this reference sheet in the AP Exam
If you looked at the reference sheet and seem to not understand some concepts here is a Khan Academy resource which can help you understand specific topics.
APCSP Create Task
Unit 3.10.2 (Ethan Tran)
- Traversing a list is the process of visiting each element in a list in a sequential order. It can be used to access, search for, and modify elements in the list.
Traversing Lists
1) Complete Traversal: All elements in a list are assessed
2) Partial Traversal: Only a given portion of elements are assessed
3) Iterative Traversal: When loops are used to iterate through a list and to access each single element at a time.
Quick Lists Reference Sheet
- A given element of a list can be evaluated using index, [ ]
1) Ex. listName[i] 2) Ex. listName[3]
- insert( ) allows a value to be inserted into a list at index i
- append( ) allows a value to be added at the end of a list
- remove( ) allows an element at index i to be deleted from a list
- length( ) returns the number of elements currently in a specific list
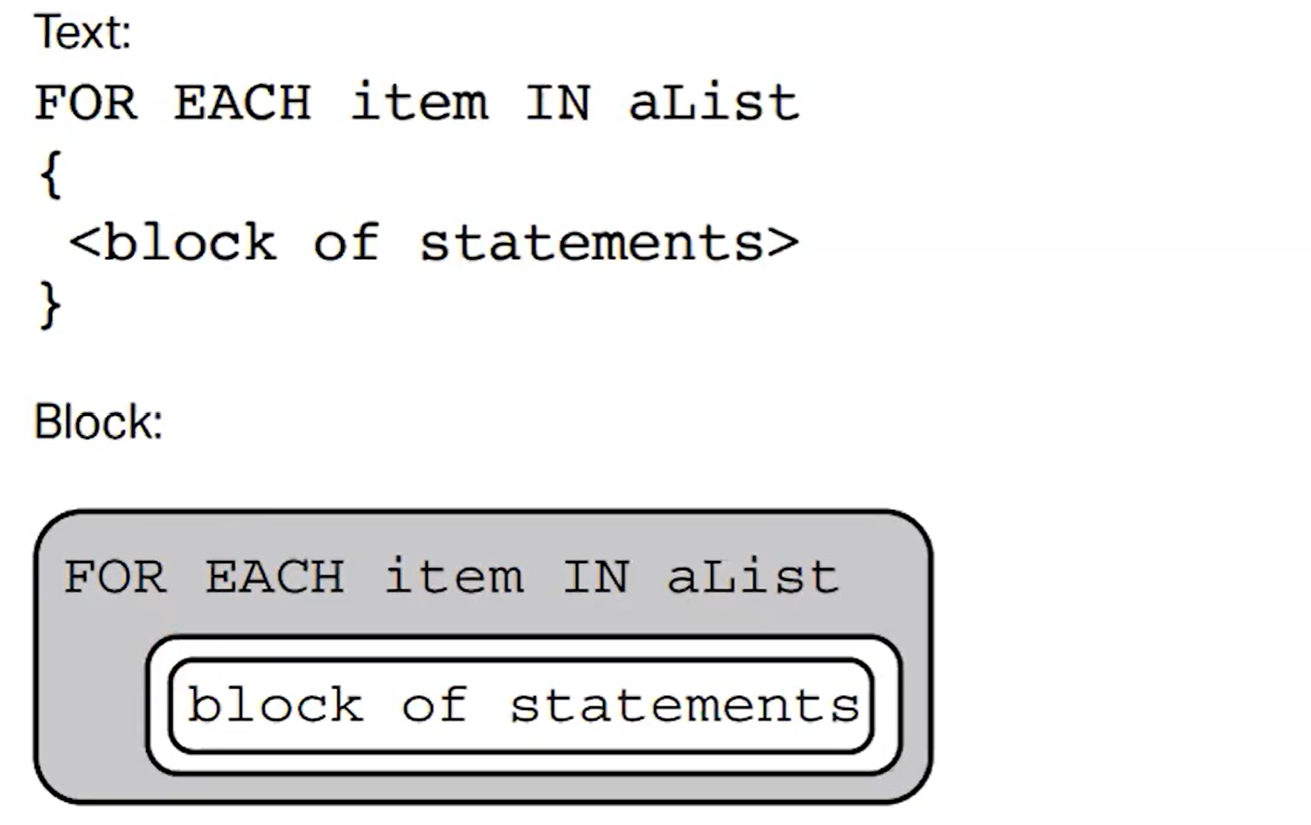
A loop such as the example above allows a user to access each item within the list
College Board Example Question
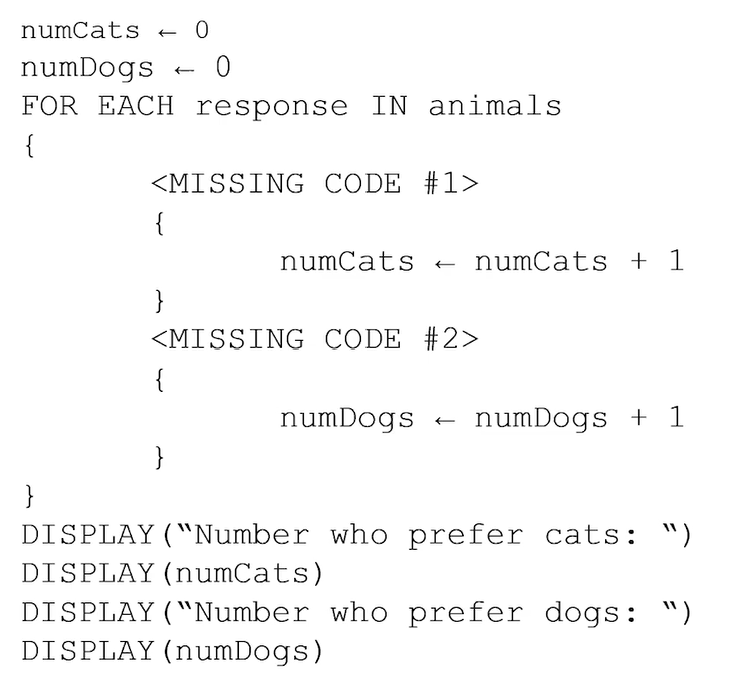
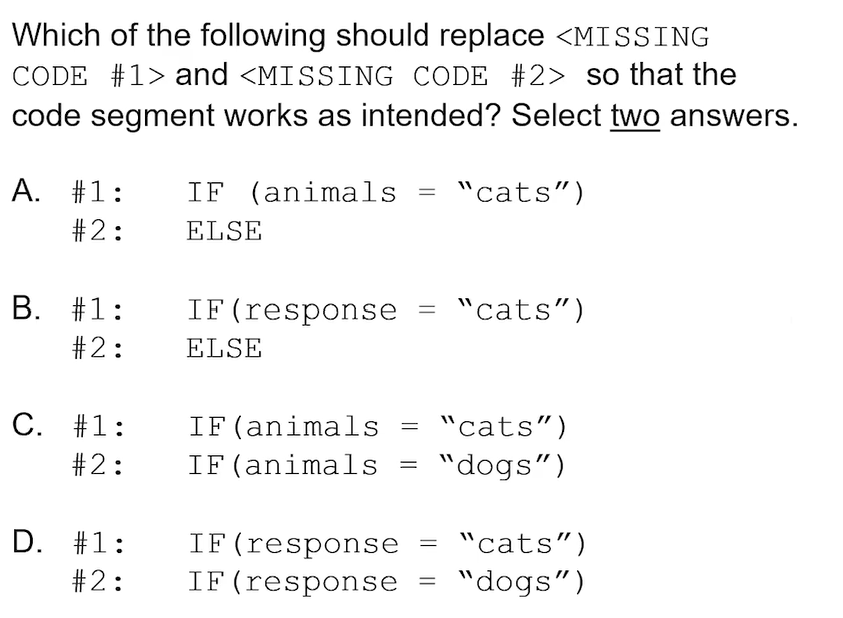
- Use the list made bellow
- Make a variable to hold the minimum and set it to potential minimum value
- Loop
- Check each element to see if it is less than the minimum variable
- If the element is less than the minimum variable, update the minimum
- After all the elements of the list have been checked, display the minimum value
nums = ["10", "15", "20", "25", "30", "35"]
print(min(nums))
import getpass, sys
import random
def ask_question (question, answer):
print(question)
ans = input(question)
print(ans)
if ans == answer:
print("Correct!")
return 1
else:
print("Wrong")
return 0
question_list = ["What allows a value to be inserted into a list at index i?" , "What allows an element at index i to be deleted from a list?" , "What returns the number of elements currently in a specific list?" , "What allows a value to be added at the end of a list?"]
answer_list = ["index()", "remove()", "length()" , "append()"]
# Set points to 0 at the start of the quiz
points = 0
# If the length of the quiz is greater than 0, then random questions will be chosen from the "question_list" set
while len(question_list) > 0:
index = random.randint(0, len(question_list) - 1)
# The points system where a point is rewarded for each correct answer
points = points + ask_question(question_list[index], answer_list[index])
# If a question or answer has already been used, then it shall be deleted
del question_list[index]
del answer_list[index]
# Calculating score using the points system and dividing it by the total number of questions (6)
score = (points / 4)
# Calculating the percentage of correct answers by multiplying the score by 100
percent = (score * 100)
# Printing the percentage, and formatting the percentage in a way where two decimals can be shown (through "{:.2f}")
print("{:.2f}".format(percent) + "%")
# Adding final remarks based upon the users given scores
if points >= 5:
print("Your total score is: ", points, "out of 4. Amazing job!")
elif points == 4:
print("Your total score is: ", points, "out of 4. Not too bad, keep on studying! " )
else:
print("Your total score is: ", points, "out of 4. Its alright, better luck next time!")